The Messenger class within MVVMLight gives developers the ability to publish an application wide event using messages that allow a subscriber to react without either the publisher or subscriber knowing anything about each other.
As always with MVVMLight it’s really simple to do.
In this example, I wanted a user to be able to click on the main menu of my application, shown on the left hand side of the screenshot below, which would update the content pane on the right hand side.
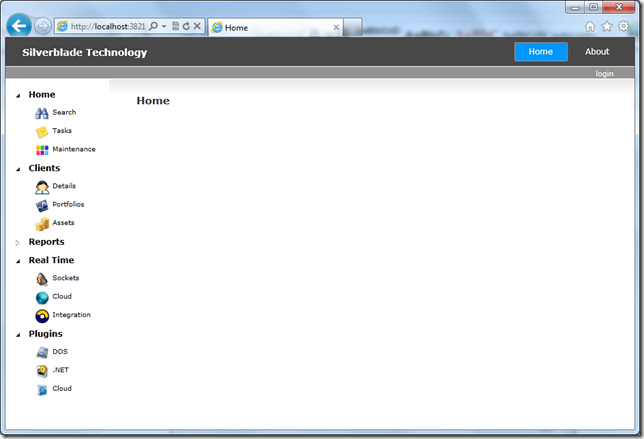
The main menu is a user control whilst the content pane is a navigation frame located within the MainPage.xaml.
To allow two pieces of code to communicate with each other, without knowing anything about each other, I will use the Messenger class in 3 simple steps.
1 Create a strongly typed message
2 Register a subscriber to the message type
Register the MainPage as the subscriber so that when the message is received the Uri within the message will be passed to the NavigateTo method to update the content pane.
3 Lastly, publish the message
When the TreeView menu is clicked the code behind checks for a Uri within the selected TreeViewItem then creates a message containing the Uri and publishes it to your application.
Summary
Using messages within your application can be a great way for your components to communicate whilst maintaining a loosely coupled architecture. This is important because you don’t have any nasty hard coded dependencies. Hard coded dependencies result in brittle, inflexible systems that can be hard to extend in the future.
The Messenger class in MVVMLight provides similar functionality to the EventAggregator class in PRISM.
You can download the WCF RIA sample application here:
http://www.stevenhollidge.com/blog-source-code/Silverblade5-WCF-RIA-MVVMLight-Messenger.zip
Please note that for demo purposes only menu options “Home > Search” and “Client > Details” have views that can be displayed within the content pane. All other menu options will return an error.