Examples of one way streaming from server to client can be found on the web but it’s pretty much always JavaScript clients. Here is a working example using the .NET stack, with WebAPI as the server and a C# console application as the client.
Download and run this WebAPI chat application which emits Server Side Events:
https://github.com/filipw/html5-push-asp.net-web-api/
I then open Visual Studio running as admin, update the code to use IIS with my machine name (Zeus) and a virtual directory, clicking the create virtual directory button, so I can track requests using Fiddler:
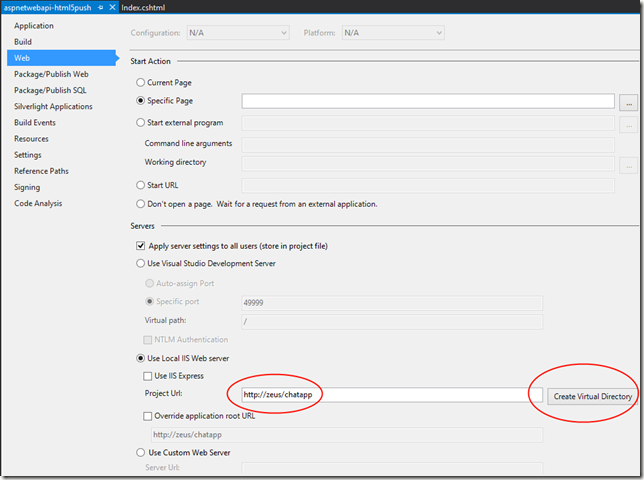
Then update the JavaScript within the app to use the same path:
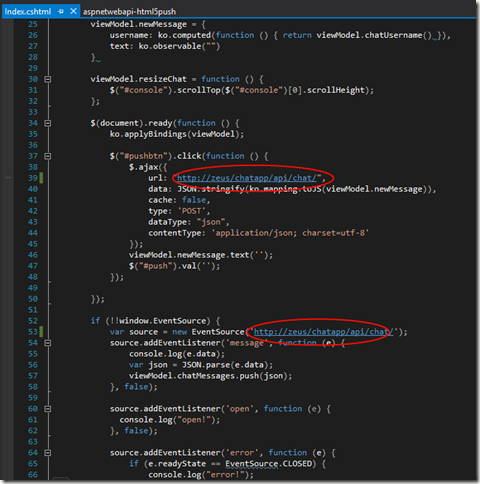
The create a Console application, using NUGET add Json.NET and paste this code in:
using System;
using System.IO;
using System.Net;
using System.Text;
using Newtonsoft.Json;
namespace ConsoleApplication2
{
class Program
{
static void Main(string[] args)
{
new WebClientWrapper();
Console.ReadKey();
}
}
public class WebClientWrapper
{
WebClient wc { get; set; }
public WebClientWrapper()
{
InitialiseWebClient();
}
// When SSE (Server side event) occurs this fires
private void OnOpenReadCompleted(object sender, OpenReadCompletedEventArgs args)
{
using (var streamReader = new StreamReader(args.Result, Encoding.UTF8))
{
var cometPayload = streamReader.ReadLine();
var jsonPayload = cometPayload.Substring(5);
var message = JsonConvert.DeserializeObject<Message>(jsonPayload);
Console.WriteLine("Message received: {0} {1} {2}", message.dt, message.username, message.text);
InitialiseWebClient();
}
}
private void InitialiseWebClient()
{
wc = new WebClient();
wc.OpenReadAsync(new Uri("http://zeus/chatapp/api/chat/"));
wc.OpenReadCompleted += OnOpenReadCompleted;
}
}
public class Message
{
public string username { get; set; }
public string text { get; set; }
public string dt { get; set; }
}
}
You’ll need to update the code for your machine name.
Now run Fiddler, the WebAPI project and the console app and add a message in the chat window:
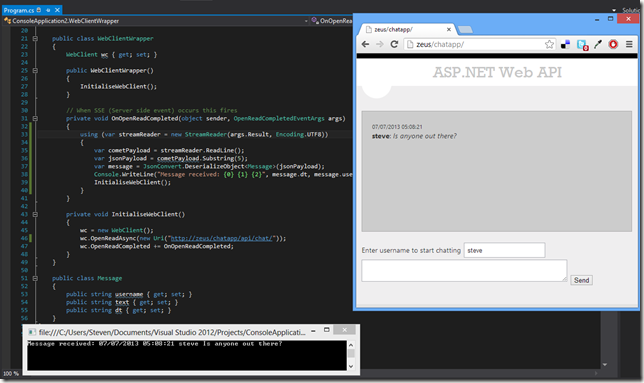
In Fiddler, select the request your console just made and select Raw, you’ll see nothing. Now right click and select COMETPeek and you’ll see the payload that was streamed.
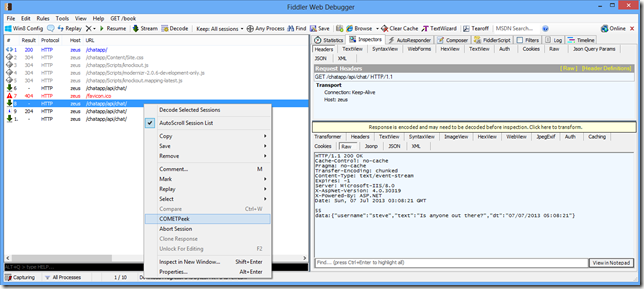